What is an API call?
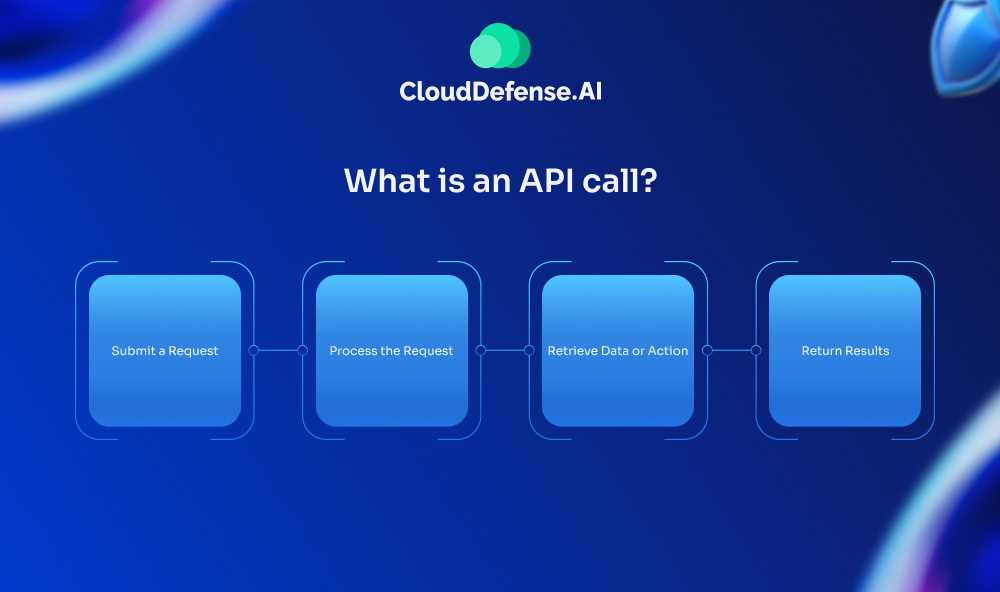
An API call, or Application Programming Interface call, is a structured request sent from one software application to another, asking for data or to execute a specific function. This process allows different systems or programs to communicate seamlessly and share information in real time.
In simple terms, an API call enables applications to:
- Submit a Request: The client application makes a request for data or an action via the API.
- Process the Request: The API translates and sends this request to the external server or program.
- Retrieve Data or Action: The server processes the request, gathers the requested information, or performs the required action.
- Return Results: The API relays the server’s response back to the client application.
So, every time you refresh your weather app, post on social media, or use online banking, you’re unknowingly making API calls! This simplified interaction is the backbone of many services that make the internet, and your apps work seamlessly.
How to Make an API Call?
To make an API call, understanding its key components ensures successful communication between client and server. Here’s a breakdown of each element:
Endpoint:
The endpoint is the specific URL where the client sends its request, pinpointing the resource or action required. For instance, an endpoint to fetch a user list might look like /users.
Method:
HTTP methods define the action the client intends to perform on the resource:
- GET: Retrieve data.
- POST: Create new data.
- PUT/PATCH: Update existing data.
- DELETE: Remove data. These methods are defined in the first line of the request message.
Headers:
Headers contain key-value pairs offering details about the request:
- Content-Type: Indicates the format of the request data (e.g., JSON, XML).
- Authorization: Contains credentials if the API requires authentication. Headers are typically included at the top of the request message.
Request Body:
Used in POST and PUT requests, the request body contains the data to be sent to the server. The data format is specified in the “Content-Type” header (e.g., JSON).
Response:
After processing the request, the server responds with:
- Status Code: A three-digit code indicating request success or error (e.g., 200 for success, 404 for not found).
- Headers: Information about the response, like content type.
- Body: Contains the requested data or details of the operation result.
These components work together to facilitate a complete API call, ensuring clear communication between client and server.
API Call Example
Here is an example of an API call to get a list of users:
Request:
GET /users HTTP/1.1
Host: api.example.com
Content-Type: application/json
{
"limit": 10,
"offset": 0
}
Response:
HTTP/1.1 200 OK
Content-Type: application/json
[
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
},
{
"id": 2,
"name": "Jane Doe",
"email": "jane.doe@example.com"
}
How do API Calls Work?
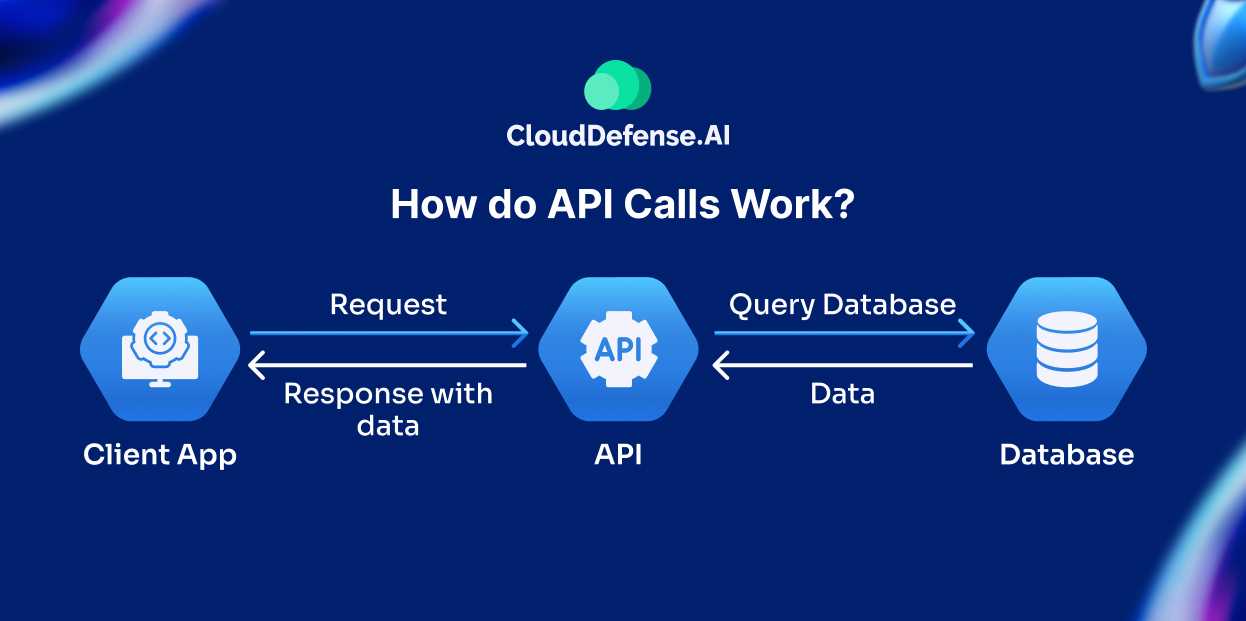
API calls enable communication between client applications and servers, allowing users to access data and perform actions. Here’s how a typical API call functions:
- User Initiates Request:
The process begins when a user performs an action in a client application, like searching for a product or booking a service.
- Client App Makes API Request:
Based on the user’s action, the client application formulates an API request containing relevant details. This request is then sent to the API endpoint.
- API Receives and Validates the Request:
Upon receiving the request, the API performs several checks:
- Validates that the request is correctly formatted and includes required data.
- Authenticates the identity of the client app (typically via an API key or other credentials).
- Authorizes the request, ensuring the client app has permission to perform the action.
- API Queries the Database:
If valid and authorized, the API sends a request to the database, translating the user’s original action into a database-friendly query.
- Database Processes Request and Retrieves Data:
The database executes the query, processes the request, and retrieves the relevant data, such as product details or user information.
- Database Sends Data Back to the API:
The database then returns the retrieved data to the API for further processing.
- API Processes and Prepares the Response:
The API may reformat or transform the data to match the client’s needs, preparing it for return.
- API Sends Response to Client Application:
The API sends the processed data back to the client application in a structured API response.
- Client App Receives and Processes the Response:
The client app receives the data, extracts relevant information, and prepares it for display.
- Client App Displays Information to the User:
The client application updates the user interface with the requested information, completing the API call.
This sequence allows smooth data exchange, keeping user experiences smooth and dynamic.
How API Calls Can Be Used for Attacks
While API calls fuel the smooth operation of our digital world, unfortunately, they can also be exploited for malicious purposes. Hackers and attackers can leverage the structure and functionality of API calls to launch various attacks, causing harm and disruption. Here’s how API calls can be weaponized:
Unauthorized Access (API Authentication Bypass):
If an API doesn’t have proper authentication mechanisms or if there are vulnerabilities in the authentication process, attackers may attempt to bypass authentication and gain unauthorized access to sensitive data or functionality.
Injection Attacks
Similar to SQL injection in web applications, injection attacks in API calls involve inserting malicious code or payloads into API requests to manipulate data, execute arbitrary commands, or compromise the integrity of the system.
Man-in-the-Middle (MitM) Attacks
Attackers may intercept and manipulate API calls between the client and server. This can lead to unauthorized access, data tampering, or eavesdropping on sensitive information being transmitted over the network.
Cross-Site Scripting (XSS)
Attackers inject malicious scripts into API responses, tricking users into executing them, potentially stealing their data or hijacking their sessions.
DoS and DDoS Attacks
Attackers may flood an API with a large number of requests (either directly or through a botnet) to overwhelm the server, causing it to become unresponsive or unavailable. This can lead to a denial of service for legitimate users.
Security Misconfigurations
Improperly configured API permissions, inadequate logging, or exposed sensitive information in error responses can be exploited by attackers to gather information or launch more sophisticated attacks.
How to secure APIs from invalid API calls?
Securing APIs from invalid calls is crucial for protecting data, preventing attacks, and ensuring seamless functionality. Here are some key strategies to achieve this:
1. Input Validation:
- Validate all data: Implement rigorous validation checks on every parameter received in an API call. Ensure data types, formats, and lengths adhere to defined standards. Reject invalid calls immediately with informative error messages.
- Sanitize inputs: Sanitize all data to remove potentially harmful characters or code that could exploit vulnerabilities like SQL injection or cross-site scripting (XSS).
2. Authentication and Authorization:
- Strong authentication: Implement robust authentication mechanisms like multi-factor authentication (MFA) to ensure only authorized users access the API.
- Granular authorization: Define clear access control lists (ACLs) specifying which users or applications can access specific resources and actions within the API. Prevent unauthorized access to sensitive data or functionalities.
3. Rate Limiting:
- Limit requests: Set limits on the number of API calls a user or application can make within a specific timeframe. This prevents brute-force attacks and DoS attempts.
- Adaptive rate limiting: Implement adaptive rate limiting algorithms that adjust limits based on user behavior and risk profiles. This helps identify and limit suspicious activity while allowing legitimate users smooth access.
4. Input Data Sanitization:
- Escape special characters: Escape special characters like quotes, apostrophes, and backslashes that could be used for injection attacks.
- Validate data structure: Ensure data adheres to predefined formats and structures.
5. Error Handling and Logging:
- Return informative error messages: Instead of generic error messages, provide specific details about the invalid call, helping developers debug and identify potential issues.
- Log all API calls: Log all API calls, including details like timestamps, source IP addresses, and requested resources. This helps identify suspicious activity and troubleshoot issues.
6. Security Best Practices:
- Keep APIs updated: Regularly update APIs with the latest security patches to address known vulnerabilities.
- Use secure protocols: Utilize HTTPS for encrypted communication and prevent data sniffing or tampering.
- Perform security audits: Conduct regular security audits and penetration testing to identify and address potential weaknesses in your API implementation.
7. API Gateway Protection:
- Utilize API gateways: Implement API gateways as a single entry point for API calls. They can enforce security policies, perform additional validation, and route requests to appropriate services.
- Implement API throttling: Implement throttling mechanisms within the API gateway to limit the rate of incoming requests and prevent DoS attacks.
Conclusion: API Security Made Easy with CloudDefense.AI
APIs are critical yet vulnerable assets in modern applications. CloudDefense.AI’s advanced API security solution offers smooth, continuous scanning to detect and address vulnerabilities, including OWASP Top 10 threats. With a pre-packaged image, CloudDefense.AI deploys directly into runtime in just two minutes—no extra setup required.
Supporting Python, Java, JavaScript, and more, CloudDefense.AI ensures broad compatibility across development environments. Its user-friendly interface simplifies API protection for both technical and non-technical teams.
Regular scans with CloudDefense.AI allow for real-time threat detection and swift vulnerability mitigation, ensuring the security of APIs across all applications. Trusted globally, CloudDefense.AI offers a powerful yet easy-to-use solution to strengthen API security with minimal effort. Check out CloudDefense.AI’s superiority through our free demo!