We know how important it is to secure applications while maintaining speed in the application development space. DevSecOps offers the perfect solution by embedding security directly into the DevOps lifecycle, allowing teams to stay agile without compromising protection.
Modern application development is getting complex as new technology is introduced, this makes it important to adopt DevSecOps best practices. Read on as we walk you through eleven best practices for implementing DevSecOps and building a security-first culture in 2025.
Before we dive into the best practices, let’s get a brief overview of DevSecOps and its core concept. Let’s get started!
What is DevSecOps?
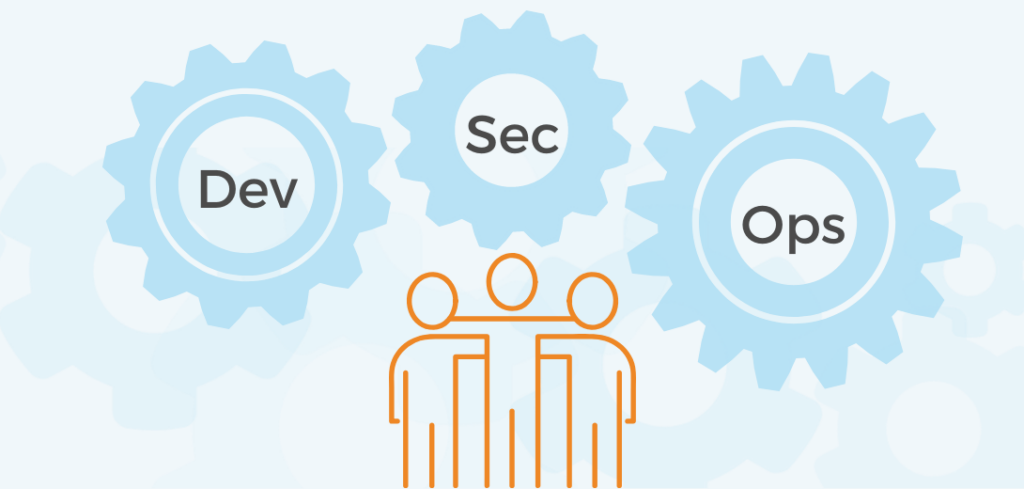
DevSecOps is an approach that embeds security throughout the entire DevOps process. As the pace of software development accelerates, DevSecOps ensures that security is a core focus, not an afterthought.
By integrating security early in the software development lifecycle, teams can address vulnerabilities more actively and reduce risks and improve efficiency.
Why is DevSecOps Important?
DevSecOps is vital because it integrates security directly into every phase of the software development lifecycle, ensuring that vulnerabilities are identified and addressed early. By embedding security into the DevOps process, teams can build safer applications without sacrificing speed or efficiency.
This approach reduces the risk of security breaches, lowers remediation costs, and fosters a culture of shared responsibility between developers, operations, and security teams. As cyber threats become more complex with each passing day DevSecOps plays a major role in maintaining the integrity and resilience of modern applications.
DevSecOps Best Practices Checklist
Implementing DevSecOps successfully requires more than just tools—it’s about adopting the right practices to secure your software from the ground up. In this checklist, we’ll cover 11 essential DevSecOps best practices that will help you integrate security smoothly into your DevOps pipeline.
From shifting security left to automating key processes, these strategies ensure your development cycles stay secure without slowing down innovation. Let’s dive into the must-have practices for building a robust DevSecOps framework.
DevSecOps Operational Best Practices
1. Build Bridges Between Teams
Collaboration is at the heart of DevSecOps. Breaking down silos between development, security, and operations teams can help ensure that everyone is working toward the same goal: secure, high-quality software.
In the traditional model, security was often viewed as an obstacle to fast development. DevSecOps changes that narrative by encouraging teams to work together, fostering a culture where security is integrated into every decision.
Teams that communicate openly can identify potential security risks earlier and collaborate on solutions. Encourage regular discussions, knowledge sharing, and joint problem-solving to build a strong foundation of trust and cooperation.
2. Set Up Real-Time Security Monitoring
Security isn’t something you can implement once and forget about. Real-time monitoring allows your team to eject security threats as they occur, providing the visibility needed to respond swiftly. This practice involves monitoring system logs, network traffic, and application performance for any anomalies that could indicate a security breach.
Real-time monitoring is like having a security camera constantly watching your application’s perimeter. If something suspicious happens, you’ll be alerted immediately, giving you the chance to respond before the issue escalates.
3. Create a Security-First Culture
At its core, DevSecOps is about building a culture where security is everyone’s responsibility. This means encouraging developers, operations teams, and security professionals to work together toward a common goal. Regular security training, open communication, and the right tools can empower your team to embrace security as a core value.
Building a security-first culture goes beyond tools and processes—it’s about mindset. When security becomes an ingrained part of your organization’s DNA, you’ll find that it becomes second nature for everyone involved.
Software Development and Deployment Best Practices
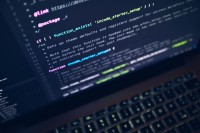
4. Start Security from Day One: The Shift Left Approach
Security is most effective when introduced early in the development process. Often referred to as “shifting left,” this practice integrates security from the very beginning, at the planning and design stages.
Imagine building a house: you wouldn’t wait until after construction to install locks on the doors and windows. Similarly, addressing security early helps prevent vulnerabilities from becoming embedded in the code, saving time, effort, and resources.
Here’s a YAML code that demonstrates a CI/CD pipeline configuration where automated security scanning is integrated early on in the development process.
# Sample of CI/CD pipeline configuration that Emphasizes Shift Left Security
pipeline:
stages:
- code_quality
- install_dependencies
- build
- test
- security_scan
- deploy
code_quality:
stage: code_quality
script:
- echo "Running static code analysis..."
- eslint . # Example static code analysis for JavaScript/Node.js
allow_failure: false # Fail the pipeline if code quality checks fail
install_dependencies:
stage: install_dependencies
script:
- echo "Installing dependencies..."
- npm install
build:
stage: build
script:
- echo "Building the application..."
- npm run build
test:
stage: test
script:
- echo "Running unit tests..."
- npm test
security_scan:
stage: security_scan
script:
- echo "Running early security checks with SAST..."
- clouddefense scan --type sast --path ./ # CloudDefense.AI SAST scanning
when: always
deploy:
stage: deploy
script:
- echo "Deploying application to production..."
only:
- main
Shifting security left empowers developers to take ownership of security. When they are trained to identify and fix security issues during coding, it fosters a proactive mindset that reduces the need for last-minute fixes before deployment.
5. Implement Continuous Security Testing
Gone are the days when security testing was reserved for the final stages of the development cycle. Continuous testing ensures that security remains a focus throughout the entire process. This practice involves scanning your code for vulnerabilities every time there’s a change—whether that’s a code commit or a deployment.
Continuous testing allows you to detect vulnerabilities in real-time, giving you the opportunity to address issues immediately. It’s like regular health check-ups: you’re more likely to catch problems early and treat them before they turn into major issues.
6. Develop and Enforce Secure Coding Standards
One of the biggest challenges in secure development is ensuring that all team members adhere to the same coding standards. Establishing secure coding guidelines helps developers write code that is resistant to vulnerabilities. These guidelines serve as a blueprint, helping developers avoid common security pitfalls like SQL injection or cross-site scripting (XSS).
Secure coding standards also promote consistency. When developers across the organization follow the same principles, it becomes easier to identify and fix vulnerabilities, creating a smoother workflow and improving the overall quality of the code.
The following Python code snippet highlights secure coding through email input validation. By ensuring that user input follows a valid email format, it helps prevent malformed data from compromising security, contributing to a strong, secure development foundation:
# Secure Coding Sample
import re
def validate_email(user_email):
"""
Validates user email to ensure it follows a standard email format.
Prevents malformed or potentially dangerous input.
"""
email_regex = r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$'
if not re.match(email_regex, user_email):
raise ValueError("Invalid email: Please enter a valid email address.")
return user_email
# Example Usage
try:
user_email = input("Enter your email: ")
valid_email = validate_email(user_email)
print(f"Email {valid_email} is valid.")
except ValueError as e:
print(e)
7. Adopt a Risk-Based Approach to Security
Not all vulnerabilities pose the same level of risk. A key principle of DevSecOps is prioritizing risks based on their potential impact. It’s essential to evaluate which vulnerabilities are critical and which can be addressed later. This risk-based approach allows teams to focus their efforts where they’re needed most.
Imagine you’re a firefighter responding to multiple calls. You’d prioritize the building with the biggest fire and greatest risk to life. Similarly, risk-based security helps you allocate resources efficiently, reducing the chances of critical vulnerabilities going unnoticed.
DevSecOps Best Practices for Using Security Tools
8. Use Automation for Efficiency
Manual security checks can slow down the process right now when rapid development is booming. Automated tools for code analysis, vulnerability scanning, and testing can identify potential security risks without disrupting the workflow. It is a safety net that catches potential issues before they impact production.
Automation is also key to scalability. As organizations expand and deploy more applications, manual testing becomes increasingly impractical. With automated security scans, teams can stay ahead of the curve, ensuring consistent, continuous protection.
9. Incorporate Security Tools in the CI/CD Pipeline
In DevSecOps, your CI/CD pipeline is the backbone of your development process. Integrating security tools into this pipeline is essential to ensuring that your code is continuously evaluated for security risks. Tools like SAST, DAST, and SCA can be added to the pipeline to detect issues early.
By doing this, you create a smooth process where security checks become a natural part of development. Security is no longer a hurdle that teams have to jump over—it’s woven into the fabric of your pipeline.
10. Use Infrastructure as Code (IaC)
Infrastructure as code (IaC) revolutionizes how infrastructure—such as servers, databases, networks, and load balancers—is managed by defining it through code. With IaC, infrastructure configurations are maintained using the same version control and practices that DevOps teams use for application code.
This approach enables teams to enforce security best practices, automate compliance checks, and adapt swiftly to evolving security requirements. Moreover, IaC enhances disaster recovery by ensuring that infrastructure configurations can be replicated and restored consistently, minimizing downtime and maintaining security integrity.
Below is a YAML example that illustrates how IaC can be used to define secure infrastructure, including features like firewall configurations and automated security patching:
infrastructure:
resources:
- name: "web-server"
type: "virtual_machine"
properties:
image: "ubuntu-20.04"
size: "Standard_DS2_v2"
network_interface: "web-nic"
enable_auto_patch: true
firewall:
rules:
- name: "allow-https"
protocol: "tcp"
port: 443
action: "allow"
description: "Allow HTTPS traffic"
- name: "allow-ssh"
protocol: "tcp"
port: 22
action: "allow"
description: "Allow SSH access"
- name: "deny-all"
protocol: "all"
action: "deny"
description: "Deny all other traffic"
automation:
- name: "auto-patching"
type: "patch_management"
schedule: "weekly"
apply_during_maintenance_window: true
backup:
- name: "daily-backup"
type: "snapshot"
schedule: "daily"
retention_days: 30
11. Treat Security Policies as Code
Security policies are often written as guidelines, but in a DevSecOps environment, they can be more powerful when treated as code. By defining security policies programmatically, you ensure that they are automatically enforced across your CI/CD pipeline. This eliminates the need for manual checks and ensures that all security policies are consistently applied.
Imagine writing a recipe that not only tells you how to bake a cake but also automatically adjusts the oven and measures the ingredients for you. Policy as code works in a similar way, making sure security is baked into every step of your process.
Conclusion: Supercharge Your DevSecOps Strategy
When it comes to DevSecOps, CloudDefense.AI stands out as a comprehensive, AI-powered security solution. It seamlessly integrates into every stage of the development lifecycle, embedding security from the ground up:
Proactive Security Right from Code to Deployment
- Complete Vulnerability and Misconfiguration Detection: CloudDefense.AI integrates smoothly with popular CI/CD tools like Jenkins, GitLab CI/CD, and GitHub Actions to scan infrastructure as code (IaC), container images, and application code for vulnerabilities and misconfigurations before deployment. By shifting security left, it ensures critical issues are addressed early, preventing last-minute fixes in production.
- Enforced Policies and Instant Remediation: Set up custom security policies that CloudDefense.AI automatically enforces across your pipeline. It flags policy violations instantly and provides remediation suggestions, making security checks part of the development process without adding delays.
Complete Visibility Across Development and Security Teams
- Unified Platform for End-to-End Security Insight: CloudDefense.AI offers a collaborative environment where both developers and security teams gain complete visibility into risks throughout the development lifecycle. This integration promotes a shared responsibility for security, facilitating stronger collaboration and more informed decision-making.
- Always-On Monitoring and Threat Detection: With CloudDefense.AI, your cloud environments are continuously monitored, identifying misconfigurations, suspicious behaviors, and potential threats in real time. This continuous vigilance ensures that your security posture is always maintained, even as changes are introduced.
Simplified Security Integration for DevSecOps Success
- Effortless Integration Without Extra Agents: CloudDefense.AI’s agentless architecture eliminates the complexity of installing and managing agents across environments. It’s a lightweight and scalable solution that simplifies security integration, minimizing overhead for teams.
- User-Centric Dashboards and Reporting Tools: The platform delivers intuitive, developer-friendly dashboards that clearly visualize risks and vulnerabilities, while offering actionable insights. The detailed reports break down security issues, enabling swift remediation while enhancing productivity.
- Flexible, Developer-Friendly Integrations: CloudDefense.AI integrates with a variety of developer tools, container registries, and project management systems, making it a natural extension of your existing DevSecOps workflows. This flexibility enhances security without slowing down innovation.
CloudDefense.AI secures every aspect of your development lifecycle, ensuring that vulnerabilities are mitigated long before they reach production.
All-in-one Application Security Suite
To fully secure your development pipeline, a single security tool isn’t enough. You need a combination of solutions to stay in line with DevSecOps best practices:
- SAST for code vulnerabilities
- DAST for real-world testing
- SCA for managing third-party risks
- IaC scanning to secure your infrastructure.
With CloudDefense.AI, you get all these tools in one platform, simplifying DevSecOps and providing complete security throughout the software lifecycle. By integrating these best practices into your DevSecOps strategy, security becomes a driving force behind innovation, not a bottleneck. Schedule a demo today and witness our superiority firsthand!